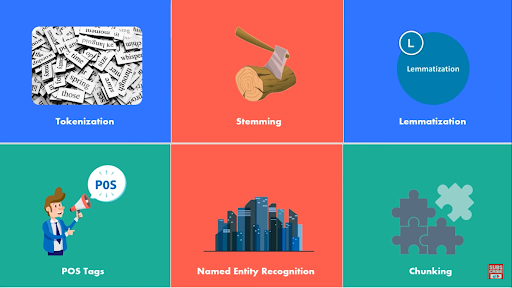
Description:-
A chatbot is a computer program that simulates human conversation through text or voice interactions. Chatbots are increasingly used in applications such as customer service, marketing, and education. They can be used to answer simple questions, provide customer support, and even interact with other applications.
In this tutorial, we will learn how to create an AI chatbot in Python using the Natural Language Toolkit (NLTK) library. NLTK is a leading platform for building Python programs to work with human language data.
What is Lemmatization?
Lemmatization is the process of grouping together the inflected forms of a word so they can be analysed as a single item. It is quite similar to stemming, with the only difference that lemmatization looks beyond word reduction, and considers a language’s full vocabulary to apply a morphological analysis to words. This results in the lemma of the word which is the dictionary form of the word, and typically the base form of the word.
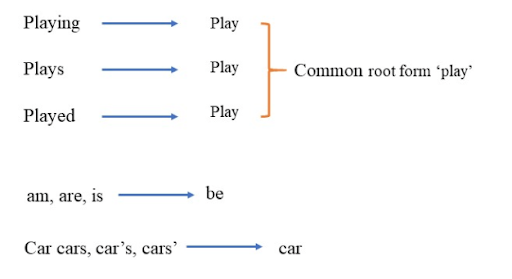
How to Create AI Chatbot In Python Using NLP (NLTK)
Step 1 – import lemmatizer function
Import necessary lemmatizer from nltk.stem wordnet lemmatizer.
Step 2 – find stem root words
Define an example_sentence for finding stem root words by lemmatizer.
output:-
Step 3. Initialize WordnetLemmatizer() function.
Find stem root words in filter sentence words
Note: In the above output, you must be wondering why no actual root form has been given for any word, this is because they are given without context. You need to provide the context in which you want to lemmatize which is the parts-of-speech (POS). This is done by giving the value for pos parameter in wordnet_lemmatizer.lemmatize.
Output:-
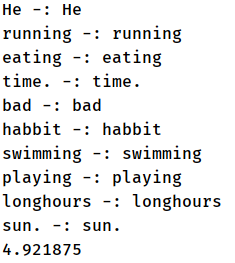
Applications of Stemming and Lemmatization:
Stemming and Lemmatization are themselves forms of NLP and widely used for:
Text Classification: Stemming and lemmatization help to reduce the size of the feature space and so, speed up the process of text classification.
Information Retrieval: Stemming and lemmatization are used for efficient information retrieval in search engines and other applications.
Natural Language Processing: Stemming and lemmatization are used for natural language processing tasks such as part-of-speech tagging, parsing, and machine translation.
Spell Checking: Stemming and lemmatization are used for spell checking and other related tasks.
POS(Part of Speech)
POS stands for Part of Speech. It is a category of words that are defined according to their syntactic functions in a sentence. There are eight main parts of speech: nouns, pronouns, verbs, adjectives, adverbs, prepositions, conjunctions, and interjections. Every word in a sentence is associated with grammar relations and a part of speech (pos) tag (nouns, verbs, adjectives, adverbs etc).
Part of speech tags are labels assigned to words in a sentence for the purpose of indicating the part of speech of the word. Common tags include noun, verb, adjective, adverb, pronoun, preposition, conjunction, and interjection.
Step 5. Import POS tags from nltk. Also, initialize POS tags.
Step 6. Define a sample string for tokenization and give POS tags to each word.
CC coordinating conjunction
CD cardinal digit
DT determiner
EX existential there (like: “there is” … think of it like “there exists”)
FW foreign word
IN preposition/subordinating conjunction
JJ adjective ‘big’
JJR adjective, comparative ‘bigger’
JJS adjective, superlative ‘biggest’
LS list marker 1)
MD modal could, will
NN noun, singular ‘desk’
NNS noun plural ‘desks’
NNP proper noun, singular ‘Harrison’
NNPS proper noun, plural ‘Americans’
PDT predeterminer ‘all the kids’
POS possessive ending parent’s
PRP personal pronoun I, he, she
PRP$ possessive pronoun my, his, hers
RB adverb very, silently,
RBR adverb, comparative better
RBS adverb, superlative best
RP particle give up
TO, to go ‘to’ the store.
UH interjection, errrrrrrrm
VB verb, base form take
VBD verb, past tense took
VBG verb, gerund/present participle taking
VBN verb, past participle taken
VBP verb, sing. present, non-3d take
VBZ verb, 3rd person sing. present takes
WDT wh-determiner which
WP wh-pronoun who, what
WP$ possessive wh-pronoun whose
WRB wh-adverb where, when
Output:-
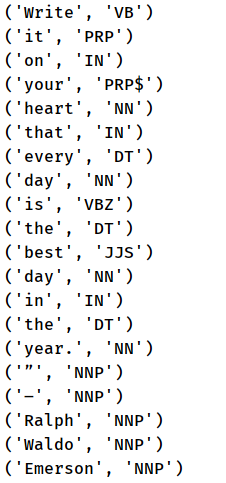
Conclusion:-
With this, you have successfully created an AI Chatbot in Python using NLTK. Your chatbot is now ready to interact with users and engage in natural conversations. With some additional training and tweaking, you can make your chatbot even smarter and more conversational. Do let us know if you have tried creating your chatbot with the stepwise guide provided here and share your experience with it. Have a great time conversing with it!
Learn more about chatbots –Creating AI Virtual Mouse Using Hand Gesture Recognition
For more such content visit – LearningBix
Leave A Comment